Open-Source
Form Framework for Vue
Vueform is a comprehensive form framework for Vue that makes form development a breeze.
form builder
An advanced tool for developing forms in Vue.js
Vueform supercharges and standardizes the entire form building process and takes care of everything from rendering to validation and processing.
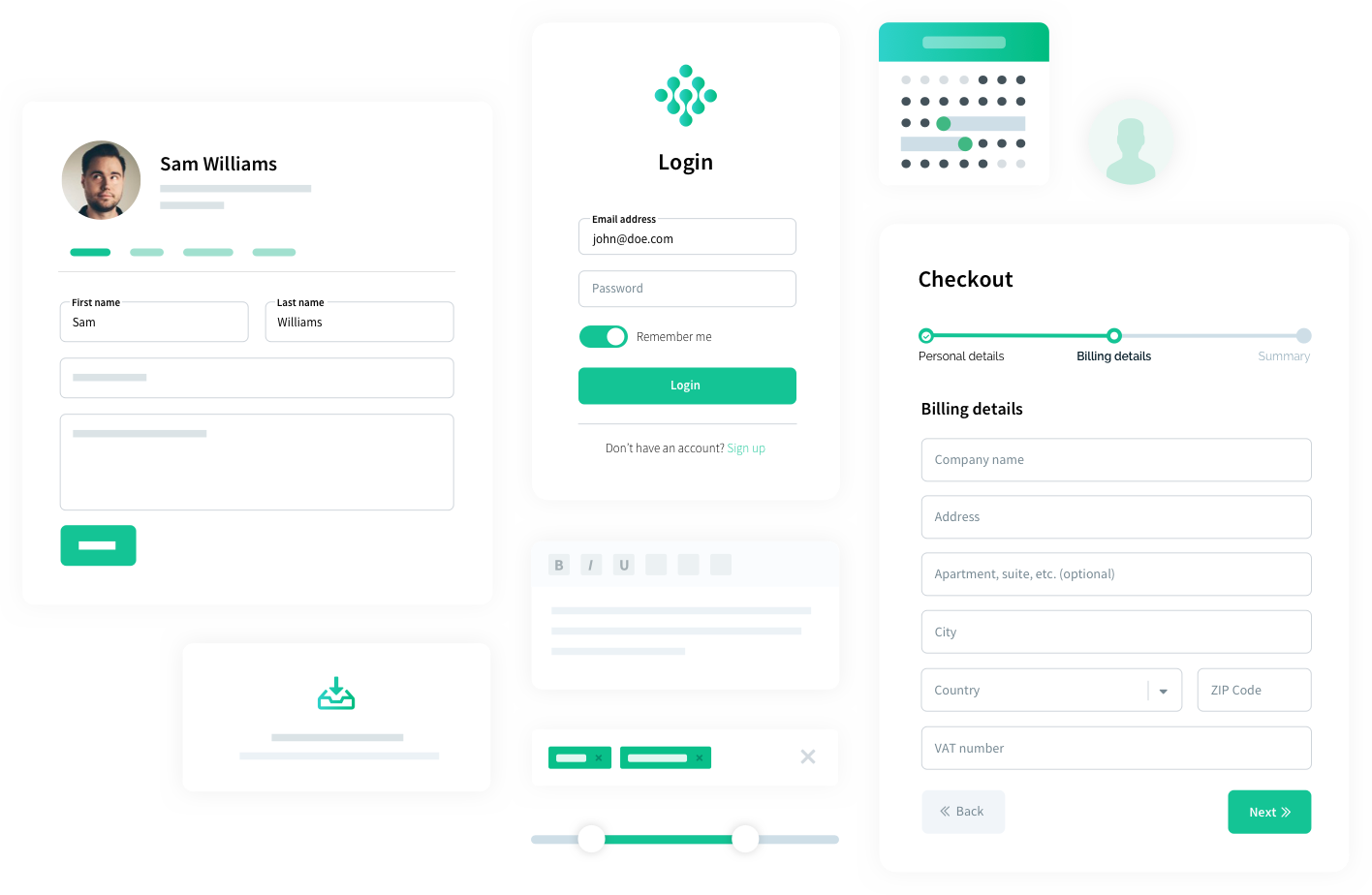
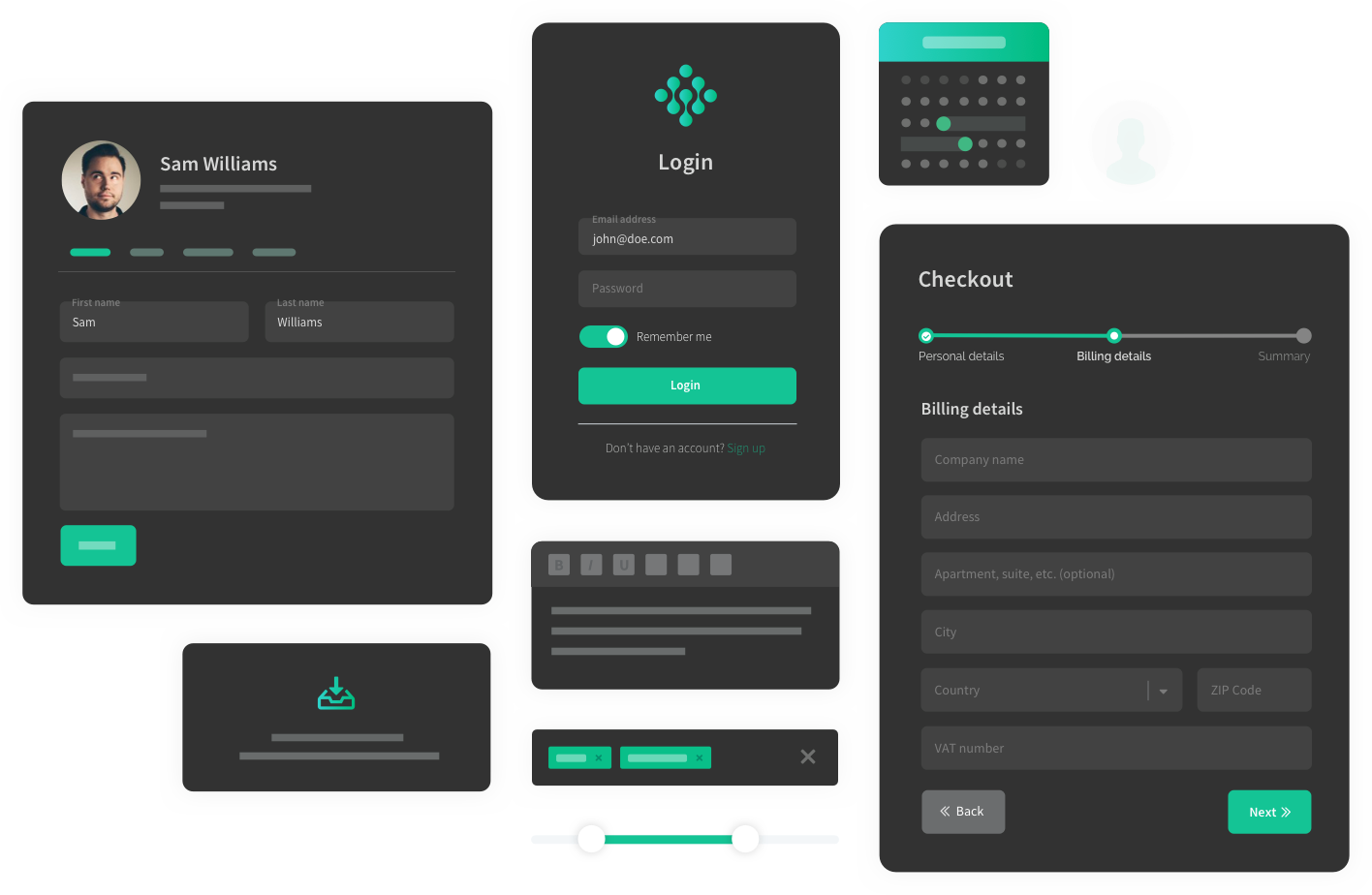
All the elements you need at your fingertips
File uploads? Date pickers? Tagging or slider? We've got you covered! No more need to hunt for elements on Github and trying to make them work. With Vueform you get everything out of the box that can be easily customized.
Check out all elements<template>
<Vueform>
<TagsElement name="tags"
:search="true"
:items="['Tailwind', 'Bootstrap', 'Bulma', '...']"
/>
<SliderElement name="slider"
:max="1000"
:merge="100"
:format="{ prefix: '$', decimals: 2 }"
/>
<PhoneElement name="phone"
allow-incomplete
/>
<DatesElement name="dates"
mode="range"
/>
<ToggleElement name="toggle">
Receive notifications
</ToggleElement>
<MultifileElement name="multifile"
view="image"
:file="{ softRemove: true }"
/>
</Vueform>
</template>
Handle nested data structures with ease
Creating nested data structures in forms can be cumbersome. Vueform provides elements that can handle infinite nesting which guarantees you will be able to handle even the most complex use cases.
More about nested elements<template>
<Vueform>
<h3>Work history</h3>
<ListElement name="history">
<template #default="{ index }">
<ObjectElement :name="index" :replaceClasses="{ ElementLayout: { outerWrapper: 'flex-1 rounded-lg pt-8 px-4 pb-4 mb-4 shadow-box' } }">
<TextElement name="name" placeholder="Company name" />
<LocationElement name="address" placeholder="Address" />
<TagsElement name="skills" placeholder="Skills used" :search="true" :items="[...]" />
<DateElement name="from" placeholder="From" :columns="4" />
<DateElement name="to" placeholder="To" :columns="4" :conditions="[['history.*.present', false]]" />
<CheckboxElement name="present" :columns="4">
Working here
</CheckboxElement>
</ObjectElement>
</template>
</ListElement>
</Vueform>
</template>
Convert forms to JSON and JSON to forms
Everything in Vueform, including elements, validation rules or steps and be stored as a JSON string. This enables rendering dynamic forms and storing even complex forms in databases. With Vueform you can create your own form builder tool!
Learn different uses of Vueform<template>
<Vueform v-bind="$data" />
</template>
<script>
export default {
data: () => ({
endpoint: '/submit/survey/489432',
schema: {
title: { type: 'static', content: `<div class='font-semibold text-2xl'>Survey</div><p class='text-gray-500 m-0'>Please fill in this survey</p>` },
question1: { type: 'text', label: 'Your name' },
question2: { type: 'text', label: 'Your age', columns: 6 },
question3: { type: 'select', label: 'Your gender', items: ['Male', 'Female', 'X'], columns: 6 },
question4: { type: 'select', label: 'How likely are you to recommend our services?', default: 10, items: [1,2,3,4,5,6,7,8,9,10], description: '1 means very unlikely, 10 means very likely' },
button: { type: 'button', buttonLabel: 'Submit', submits: true, },
}
})
}
</script>
50+ built-in validation rules, ready to use
Vueform provides a nice set of built-in validation rules but you can create custom ones just as easily. Validators can watch each other's value, run asynchronously or even be tied to conditions.
Check out all the rules<template>
<Vueform>
<TextElement name="text" placeholder="Text"
rules="required"
/>
<TextElement name="url" placeholder="Url"
rules="url"
/>
<TextElement name="email" placeholder="Email"
rules="exists:users"
/>
<DateElement name="date" placeholder="Date"
rules="after:today"
/>
<TextElement name="password" placeholder="Password"
rules="confirmed"
/>
<TextElement name="password_confirmation" placeholder="Password again"
/>
<CheckboxElement name="terms"
rules="accepted"
>I accept the Terms of Use</CheckboxElement>
</Vueform>
</template>
Conditional rendering
Using v-if
to render certain elements conditionally is no magic. Handling that on a data level is a different thing. Vueform removes all the struggle of creating conditional forms so you can focus on the important parts.
<template>
<Vueform>
<SelectElement name="question1" :items="['Yes', 'No']" placeholder="..."/>
<SelectElement name="question2" :items="['Yes', 'No']" placeholder="..."
:conditions="[['question1', 'Yes']]"
/>
<SelectElement name="question3" :items="['Yes', 'No']" placeholder="..."
:conditions="[['question2', 'Yes']]"
/>
<SelectElement name="prize" :items="[...]" placeholder="..."
:conditions="[['question3', 'Yes']]"
/>
<StaticElement name="no_prize" :conditions="[
function(form$) {
return form$.data.question1 === 'No' ||
form$.data.question2 === 'No' ||
form$.data.question3 === 'No'
}
]">We're sorry :( You've almost made it.</StaticElement>
</Vueform>
</template>
Break forms into steps
Everybody hates filling in long forms. They might not even get started if all they see is an endless list of fields. Vueform makes it easy to break forms into steps helping your users to have a more convenient experience when using your product.
Learn more about steps<template>
<Vueform>
<template #empty>
<FormSteps>
<FormStep name="personal" :elements="['name', 'bio']"
/>Personal</FormStep>
<FormStep name="account" :elements="['email', 'password']"
/>Account</FormStep>
<FormStep name="social" :elements="['facebook', 'twitter']"
/>Social</FormStep>
</FormSteps>
<FormElements>
<TextElement name="name" label="Name" />
<TextareaElement name="bio" label="bio" rows="8" />
<TextElement name="email" label="Email" />
<TextElement input-type="password" name="password" label="Password" />
<TextElement name="facebook" label="Facebook" />
<TextElement name="twitter" label="Twitter" />
</FormElements>
<FormStepsControls />
</template>
</Vueform>
</template>
Available in 19 languages, translate contents
It can be convenient for websites operating in multiple languages to translate different parts of the published content easily. Vueform supports this by providing translatable input fields that have multiple outputs in different languages.
Learn more about i18n<template>
<Vueform multilingual>
<TTextEelement
name="title"
label="Title"
/>
<TEditorElement
name="content"
label="Content"
/>
</Vueform>
</template>

"The extensibility surprised us. We have been able to create our custom components fairly easily. Vueform and the framework it provides lets us build things on top of it quite easily. Even if the requirement is quite different from the base component that we are working on."
— Saideep Talari, CTO, SenseHawk
maximum productivity?
👋Hire Vueform team for form customizations and development
Visit siteLegal & contact
Subscribe to our newsletter